Building an Android Plugin
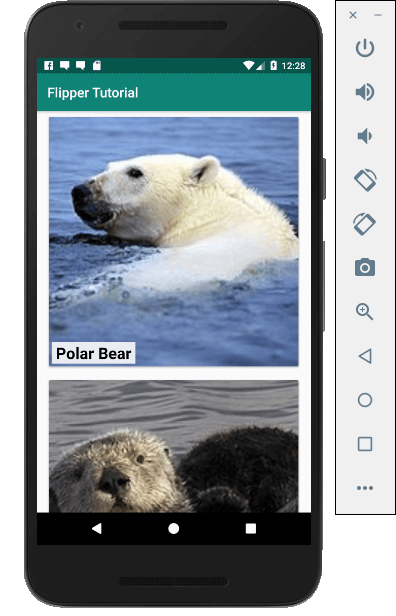
For the purpose of the tutorial, it's assumed you already have an existing Android application in which you have a feed or list of items.
Part of what makes Flipper so useful is allowing users to inspect the internals of their app. This part of the tutorial is concerned with how to make data available to the Flipper desktop app, which, for the sake of this tutorial, is called 'Sea-mammals' (an app based on sea mammals).
You can find the source code for this tutorial on GitHub.
Creating a Pluginβ
On Android, a Flipper plugin is a class that implements the FlipperPlugin interface.
The interface consists of the following four methods:
getId() -> String
- specify a unique string so the JavaScript side knows where to talk to. This must match the name attribute in thepackage.json
, which is examined later in this tutorial.onConnect(FlipperConnection)
- this method is called when the desktop app connects to the mobile client and is ready to receive or send data.onDisconnect()
- sets the connection to nil.runInBackground() -> Boolean
- unless this is true, only the currently selected plugin in the Flipper UI can communicate with the device.
The following code shows the implementation of these methods for the Sea-mammals app:
import com.facebook.flipper.core.FlipperConnection
import com.facebook.flipper.core.FlipperObject
import com.facebook.flipper.core.FlipperPlugin
import com.facebook.flipper.sample.tutorial.MarineMammals
class SeaMammalFlipperPlugin : FlipperPlugin {
private var connection: FlipperConnection? = null
override fun getId(): String = "sea-mammals"
override fun onConnect(connection: FlipperConnection?) {
this.connection = connection
MarineMammals.list.mapIndexed { index, (title, picture_url) ->
FlipperObject.Builder()
.put("id", index)
.put("title", title)
.put("url", picture_url).build()
}.forEach(this::newRow)
}
override fun onDisconnect() {
connection = null
}
override fun runInBackground(): Boolean = false
private fun newRow(row: FlipperObject) {
connection?.send("newRow", row)
}
}
The two interesting items here are onConnect
and newRow
, which sends a message to the desktop app and is identified with the same name 'newRow'.
For the sea-mammals app, there is a static data source. However, in real life, you'll likely dynamically receive new data as the user interacts with the app. So, while in the Sea-mammals app, the data is sent all at once with onConnect
, in real life, you'd set up a listener to call newRow
as new data arrives.
You may have notices that rather than sending random Object
s over the wire, FlipperObject
s are used.
A FlipperObject
works similar to a JSON dictionary and has a limited set of supported types like strings, integers and arrays.
Before sending an object from your native app to the desktop, you first need to break it down into FlipperObject
-serializable parts.
Registering your pluginβ
Now, all you need to do is let Flipper know about your new plugin. You do this by calling addPlugin
on your FlipperClient
, which is normally created at application startup.
val flipperClient = AndroidFlipperClient.getInstance(this)
// Add all sorts of other amazing plugins here ...
flipperClient.addPlugin(SeaMammalFlipperPlugin())
flipperClient.start()
Next stepβ
When starting your application, Flipper tells the desktop application about the plugin it supports (including 'Sea-mammals') and looks for a corresponding JavaScript plugin by that name.